Unity の Project TCC を触って、Unity の機能を学んでいきましょう。
Project TCC で遊ぼう~UC-01-03 Move and Jump: キャラクターにジャンプさせる~
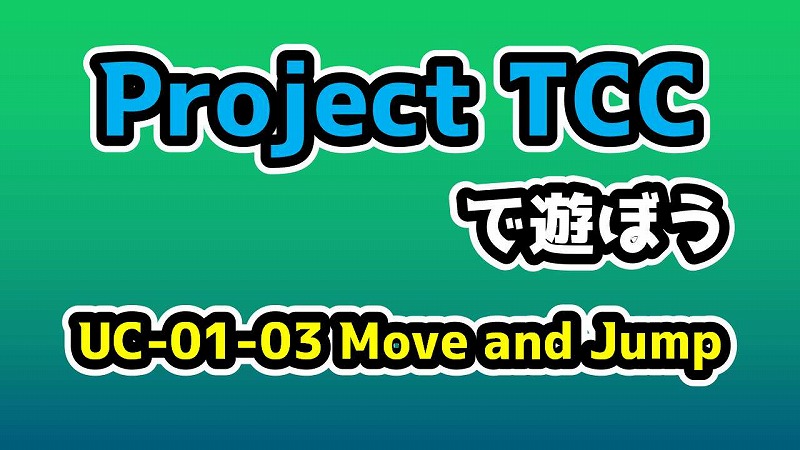
UC-01-03 Move and Jump
変更点の確認
UC-01-03_Move_and_Jump シーンを開きます。
Unityエディター上で再生して、ユニティちゃんをジャンプさせてみましょう。
UC-01-02_Walk_and_Run シーンと違うところは、Player オブジェクトの Script Machine に Jump の処理が追加されています。
Space キーを押すと、ジャンプします。
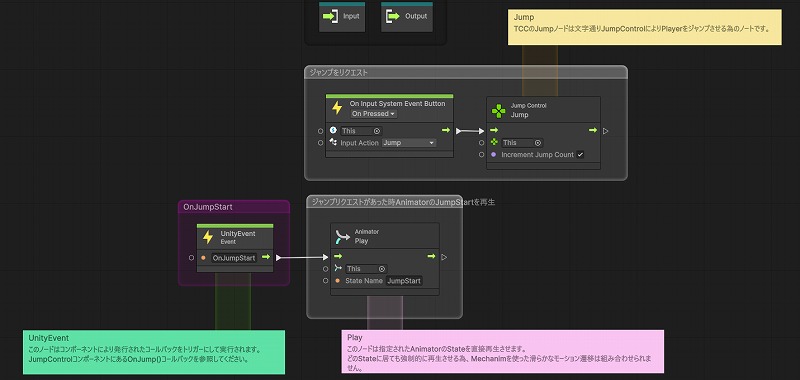
シーンの複製
UC-01-02_Walk_and_Run_Copy シーンを複製して、UC-01-03_Move_and_Jump_Copy という名前にします。
Input Action Asset の設定
Assets/TCC/Scenes/01_Move/Data_UC-01-02_Walk_and_Run_Copy フォルダを複製します。
フォルダ名は Data_UC-01-03_Move_and_Jump_Copy にします。
フォルダの中身のファイルもリネームします。
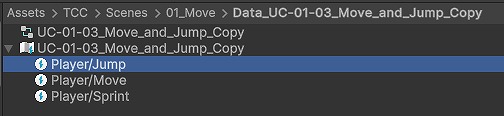
Input Action Asset(UC-01-03_Move_and_Jump_Copy)をダブルクリックします。
下記のような画面が表示されます。
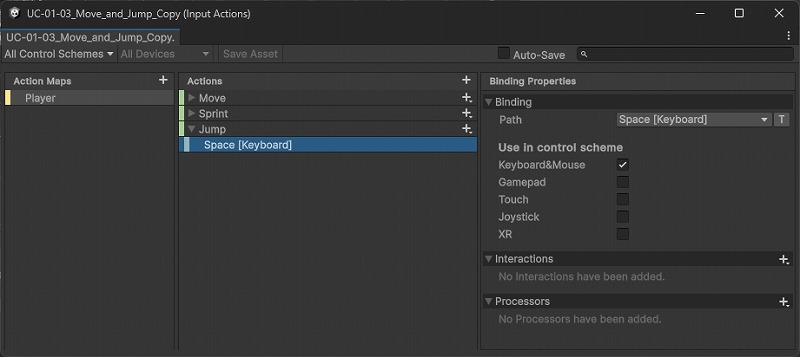
Actions の +ボタンを押して、Jump を追加します。
Action Type は Button を選択します。
Binding では Spaceキーを割り当てます。
Use in control scheme の Keyboard&Mouse に忘れずにチェックを付けましょう。
Player オブジェクトの Player Input コンポーネントの Actions に Input Action Asset を設定します。

Animator の設定
Assets/TCC/Scenes/01_Move/Data_UC-01-03_Move_and_Jump/UC-01-03_Move_and_Jump.controller を複製して、Assets/TCC/Scenes/01_Move/Data_UC-01-03_Move_and_Jump_Copy/UC-01-03_Move_and_Jump_Copy.controller にリネームします。
Player オブジェクトの Animator コンポーネントの Controller に UC-01-03_Move_and_Jump_Copy を設定します。

JumpControl コンポーネントの追加
Player オブジェクトに JumpControl コンポーネントを追加します。
一緒に Gravity と GroundCheck と HeadContactCheck コンポーネントも追加します。
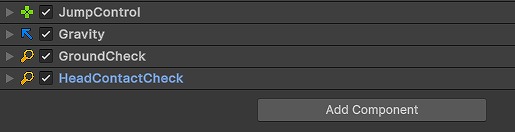
C# スクリプトの作成
Player オブジェクトにくっ付いている MyWalkTest にプログラムを追加していきます。
MyWalkTest の内容はこちらになります。
using Unity.TinyCharacterController.Check;
using Unity.TinyCharacterController.Control;
using UnityEngine;
using UnityEngine.InputSystem;
public class MyWalkTest : MonoBehaviour {
private MoveControl m_rMoveControl;
private JumpControl m_rJumpControl;
private GroundCheck m_rGroundCheck;
private Animator m_rAnimator;
private PlayerInput m_rPlayerInput;
private InputAction m_rMove;
private InputAction m_rSprint;
private InputAction m_rJump;
void Awake() {
TryGetComponent<MoveControl>(out m_rMoveControl);
TryGetComponent<JumpControl>(out m_rJumpControl);
TryGetComponent<GroundCheck>(out m_rGroundCheck);
TryGetComponent<Animator>(out m_rAnimator);
TryGetComponent<PlayerInput>(out m_rPlayerInput);
if (m_rPlayerInput != null) {
m_rMove = m_rPlayerInput.actions["Move"];
m_rSprint = m_rPlayerInput.actions["Sprint"];
m_rJump = m_rPlayerInput.actions["Jump"];
}
}
private void OnEnable() {
if (m_rJumpControl != null) {
m_rJumpControl.OnJump.AddListener(OnJumpStart);
}
if (m_rPlayerInput != null) {
m_rMove.performed += OnMove;
m_rMove.canceled += OnMoveStop;
m_rSprint.performed += OnSprint;
m_rSprint.canceled += OnSprintStop;
m_rJump.started += OnJump;
}
}
private void OnDisable() {
if (m_rJumpControl != null) {
m_rJumpControl.OnJump.RemoveListener(OnJumpStart);
}
if (m_rPlayerInput != null) {
m_rMove.performed -= OnMove;
m_rMove.canceled -= OnMoveStop;
m_rSprint.performed -= OnSprint;
m_rSprint.canceled -= OnSprintStop;
m_rJump.started -= OnJump;
}
}
private void Update() {
if (m_rMoveControl != null) {
float fSpeed = m_rMoveControl.CurrentSpeed;
if (m_rAnimator != null) {
m_rAnimator.SetFloat("Speed", fSpeed);
}
}
if (m_rGroundCheck != null) {
if (m_rAnimator != null) {
m_rAnimator.SetBool("IsGround", m_rGroundCheck.IsOnGround);
}
}
}
private void OnMove(InputAction.CallbackContext context) {
if (m_rMoveControl == null) {
return;
}
Vector2 value = context.ReadValue<Vector2>();
m_rMoveControl.Move(value);
}
private void OnMoveStop(InputAction.CallbackContext context) {
if (m_rMoveControl == null) {
return;
}
Vector2 value = context.ReadValue<Vector2>();
m_rMoveControl.Move(value);
}
private void OnSprint(InputAction.CallbackContext context) {
if (m_rMoveControl == null) {
return;
}
m_rMoveControl.MoveSpeed = 4f;
}
private void OnSprintStop(InputAction.CallbackContext context) {
if (m_rMoveControl == null) {
return;
}
m_rMoveControl.MoveSpeed = 1.2f;
}
private void OnJump(InputAction.CallbackContext context) {
if (m_rJumpControl == null) {
return;
}
m_rJumpControl.Jump(true);
}
private void OnJumpStart() {
if (m_rAnimator != null) {
m_rAnimator.Play("JumpStart");
}
}
}
Level の変更
SbS_Level_00 の代わりに SbS_Level_01 を配置しましょう。
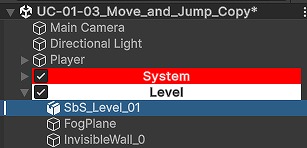
マテリアルは SbS_Level_02 に変更します。
MeshCollider コンポーネントを追加します。
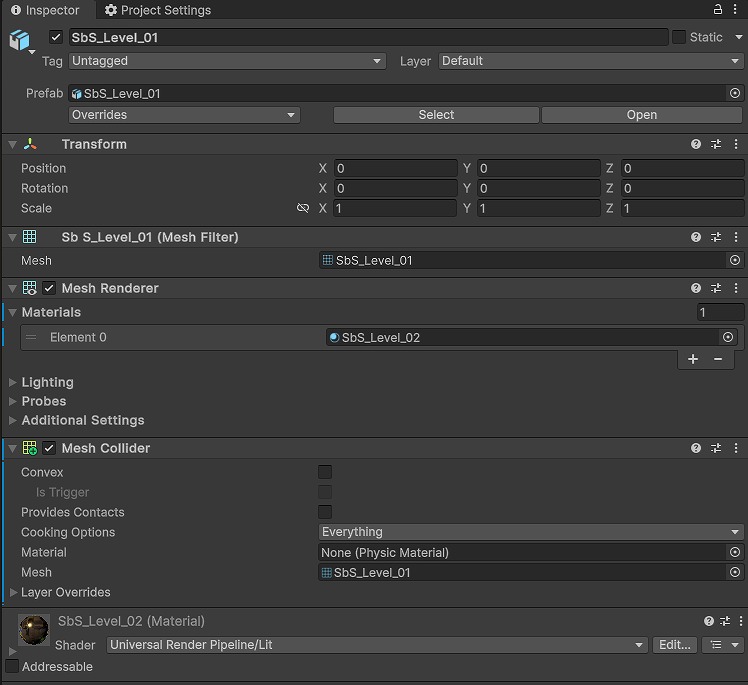
動作確認
Unityエディターで再生して、動作確認します。
ユニティちゃんをジャンプさせることが出来れば成功です。
まとめ
Project TCC のキャラクターをジャンプさせるサンプルを Visual Scripting から C# スクリプトに変換して動かしてみました。
JumpControlコンポーネントを使ってジャンプさせることが出来ます。
コメント